Cross Chain Messaging
Introduction
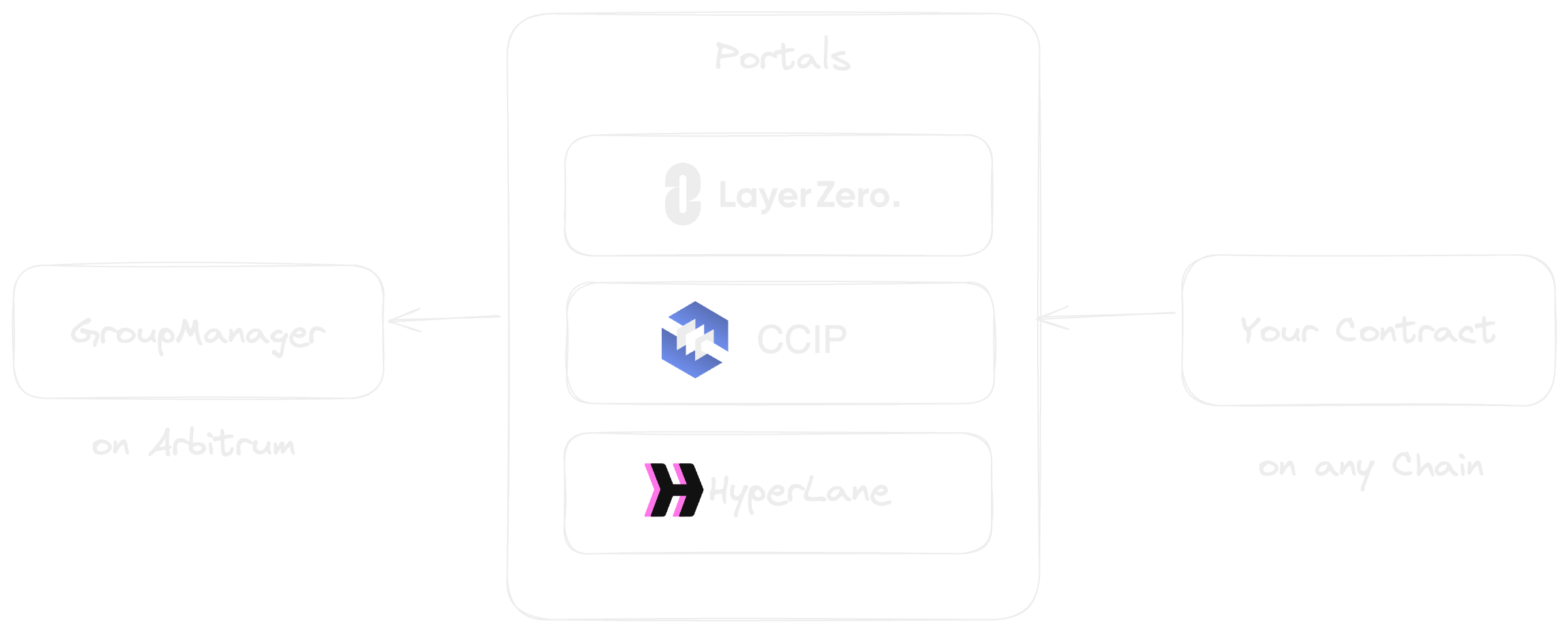
Manage Groups and Prove from any Chain.
Interface
Call the following functions on the forwarder contract to pass messages to the base chain GroupManager Contract.
Manage Groups and Prove from any Chain.
Call the following functions on the forwarder contract to pass messages to the base chain GroupManager Contract.